Hello ! First thing first, i’m quite the noob concerning python. I’m making a little project for the imminent birthday of my sister and i’m trying to do a pretty thingy : a lamp. But one who does rainbow. (That’s cool right ?)
So, that is what i wrote :
#!/usr/bin/env python
import colorsys
import time
import unicornhat as unicorn
print("Program starting...")
unicorn.set_layout(unicorn.PHAT)
unicorn.rotation(0)
unicorn.brightness(1)
unicorn.set_all(255, 0, 0)
unicorn.show()
time.sleep(60)
unicorn.set_all(255, 52, 0)
unicorn.show()
time.sleep(60)
unicorn.set_all(255, 178, 0)
unicorn.show()
time.sleep(60)
unicorn.set_all(232, 255, 0)
unicorn.show()
time.sleep(60)
unicorn.set_all(255, 52, 0)
unicorn.show()
time.sleep(60)
unicorn.set_all(255, 77, 0)
unicorn.show()
time.sleep(60)
unicorn.set_all(0, 255, 201)
unicorn.show()
time.sleep(60)
unicorn.set_all(0, 213, 255)
unicorn.show()
time.sleep(60)
unicorn.set_all(0, 125, 255)
unicorn.show()
time.sleep(60)
unicorn.set_all(4, 0, 255)
unicorn.show()
time.sleep(60)
unicorn.set_all(107, 0, 255)
unicorn.show()
time.sleep(60)
unicorn.set_all(235, 0, 255)
unicorn.show()
time.sleep(60)
unicorn.set_all(255, 0, 44)
unicorn.show()
time.sleep(60)
So… The rainbow, i kinda got it. But there is 0 smooth between colors (haven’t figured out how to do transitions) and i don’t really know how to make it loop. Can someone help me ? Thank you !
First and foremost, you should probably brush up on how colour spaces work, a great place to start (to blow my own trumpet :D) is my guide here: https://learn.pimoroni.com/tutorial/unicorn-hat/making-rainbows-with-unicorn-hat
While it’s far from the only solution, using the HSV colour space- which has only one value for actual colour- is one of the easiest ways to transition between colours. Its downfall is that you have to move through a lot of intermediate colours on your way. To transition from Green to Pink for example you either have to move through Yellow->Orange->Red or Teal->Blue->Purple.
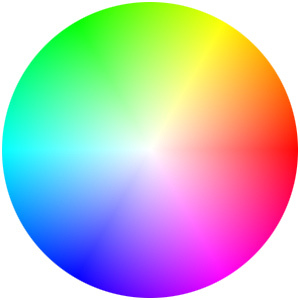
The trick to having a constantly running, smooth transition between colours is not to rely on time.sleep()
at all, but to rely on time.time()
which is the time in seconds. (since 1st January 1970 A.K.A. “The Epoch”)
This number will count up every second, so if you want to move 1 degree hue around the HSV spectrum (around the circumference of that circle pictured above) every second you need only do:
time.time() % 360
Try running python
, importing time
and typing that a few times to see what happens.
Thanks for your help ! Checking your links right now :)
(Sorry for the 2nd post)
I tried to simply run the code you gave on the tutorial but i run into some errors :
pi@raspberrypi:/home/unicorn-hat/examples/phat $ sudo ./moorg.py
./moorg.py: 1: ./moorg.py: import: not found
./moorg.py: 2: ./moorg.py: import: not found
./moorg.py: 3: ./moorg.py: import: not found
./moorg.py: 5: ./moorg.py: Syntax error: word unexpected (expecting ")")
I’m kinda lost on these functions. English is not my mother tongue nor Python and i don’t quite understand well all what’s happening.
If i can’t do pretty rainbow, no problem. I just want to light up all the led the same colors, do a little transition (if i manage to understand that) to another color, & on, & on and then repeat from the start.
I understand the basics thingy of lighting up all the led the same colors but i’m lost after that, i read a ton a scripts found on github & here but i’m still lost. :’(
You should run:
sudo python moorg.py
Unless this line is at the top of your script: #!/usr/bin/env python
then it will be run as “Bash” and not “Python”.
Oh, i see, if i even forget those basic things… And i still have no luck i guess :
pi@raspberrypi:/home/unicorn-hat/examples/phat $ sudo python moorg.py
Traceback (most recent call last):
File "moorg.py", line 13, in <module>
set_pixel_hsv(x,y,(1.0/8)*x,(1.0/4)*y,1.0)
File "moorg.py", line 9, in set_pixel_hsv
uh.set_pixel(x,y,r,g,b)
File "build/bdist.linux-armv6l/egg/unicornhat.py", line 214, in set_pixel
File "build/bdist.linux-armv6l/egg/unicornhat.py", line 183, in get_index_from_xy
TypeError: list indices must be integers, not float
Exiting cleanly
Could these errors happens because i use a pHAT & not a HAT ?
At the point you’re calling set_pixel(x,y,r,g,b)
your x, y, r, g and b values should all be integers/whole numbers.
You might get away with set_pixel(int(x), int(y), int(r), int(g), int(b))
but it’s tidier to make that conversion as early as possible.