Right, let’s learn things!
I’m quite rusty in this respect myself, so bear with me. I’m going to go back to THE BASICS!
Note: after writing all this it doesn’t look like we need to make a change to write_string
after all.
A character encoding is basically just an established standard for representing, in numbers, a particular character.
Say you and I agree that 1 = ‘A’, 2 = ‘Y’ and 3 = ‘R’. Both of us have computers that understand this encoding. In fact, let’s say its circa 1980 and they have built-in chips that do nothing but convert a number into the right character for display on screen ( for example: http://dustlayer.com/vic-ii/2013/4/23/vic-ii-for-beginners-part-2-to-have-or-to-not-have-character ).
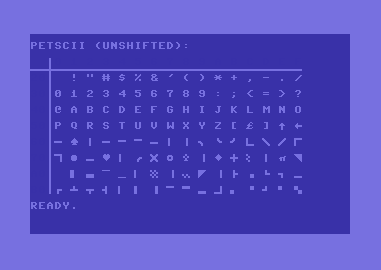
If I send your computer the string: 0x02 0x01 0x03 0x03
then it will decode these numbers by looking them up in the ‘Character ROM’ and display YARR
on your screen by drawing the pixels it finds.
Luckily hardware ROM chips have been replaced with software that’s a zillion times more complicated but still basically understands that 0x02
means “Look up how to draw a Y and display it on the screen.”
We’re going to use the Python’s unicode handling which, luckily, matches quite handily to ASCII for the first 127 codepoints and defines a bunch of useful European and mathematical symbols in the next 128 codepoints. It looks something like this (ignoring the few unprintable characters mixed in):
!"#$%&'()*+,-./0123456789:;<=>?@
ABCDEFGHIJKLMNOPQRSTUVWXYZ[\]^_` <--- WINKY FACE!
abcdefghijklmnopqrstuvwxyz
{|}~
<--- font.png ends here
¡¢£¤¥¦§¨©ª«¬®¯°±²³´µ¶·¸¹º»¼½¾¿
ÀÁÂÃÄÅÆÇÈÉÊËÌÍÎÏÐÑÒÓÔÕÖרÙÚÛÜÝÞß
àáâãäåæçèéêëìíîïðñòóôõö÷øùúûüýþ
So ideally, these are the characters we want to define in the font and be able to display on Scroll pHAT.
Perhaps the best way to do this is to update font.png in tools: https://github.com/pimoroni/scroll-phat/tree/master/tools and then update “mkfont.py” to read in and store the additional characters.
font.png runs in 3 columns of 32 characters, giving a total of 96 from codepoints 0x20 to 0x7f (or 32 to 127). These represent the visible (printable) characters of ASCII.
Using a mix of your pixel font, and some hand tweaking, we should be able to expand this font.png to include 4 new columns- another 128 codepoints- and update “mkfont.py” to read in these extra columns.
I think if the font is updated, and you pass text strings into write_string
like so:
write_string(u'Café 99º - ½ off coffee today! ¤±¤')
Then everything should be golden :D